Passing parameters into a URL is a common requirement when working with APIs or building web applications. Python’s requests
library is one of the most popular tools for making HTTP requests, and it provides an elegant way to manage query parameters when sending requests to an endpoint. Properly handling URL parameters is essential for ensuring that the server receives the correct data and responds as expected.
This article explains how to pass parameters into URLs using Python’s requests
library. Readers will learn about its syntax, best practices, and some essential tips to handle edge cases effectively.
Understanding URL Parameters
URL parameters are key-value pairs that are appended to the end of a URL to pass additional information to the server. They typically come after a question mark (?
) in the URL and are separated by ampersands (&
). For example:
https://api.example.com/users?name=John&age=30
In this URL, name
and age
are parameters, and their values are John
and 30
, respectively.
Passing Parameters with Python’s requests
Python’s requests
library allows you to pass parameters easily by using the params
parameter when making an HTTP request. Here’s how it works:
Step-by-Step Example
- Import the
requests
library:import requests
- Define the base URL: Example:
https://api.example.com/users
- Supply a dictionary of query parameters.
- Pass the parameters to the
params
argument of therequests.get()
method.
Here’s a simple example:
import requests
# Define the base URL and query parameters
url = "https://api.example.com/users"
params = {'name': 'John', 'age': 30}
# Send a GET request with parameters
response = requests.get(url, params=params)
# Print the response URL (for demonstration)
print(response.url)
# Access the response content
print(response.text)
Output of the code:
https://api.example.com/users?name=John&age=30
In the above example, the requests.get()
method automatically converts the dictionary into a query string and appends it to the end of the URL, ensuring it is properly encoded.
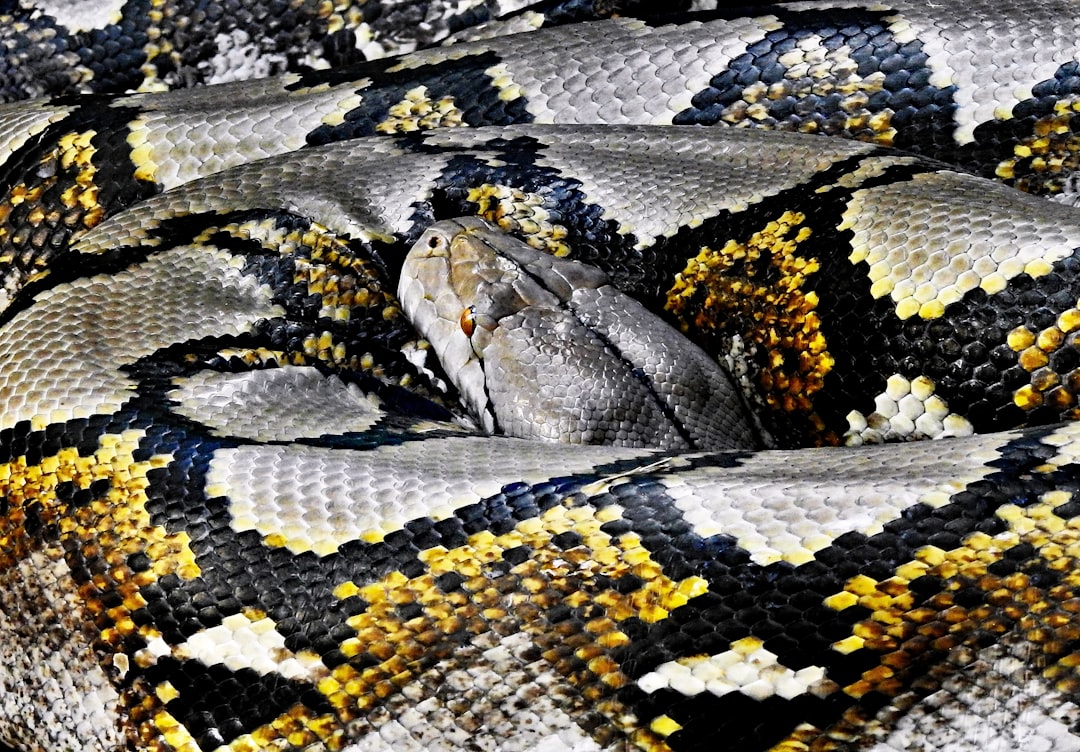
Best Practices for Passing Parameters
While using the requests
library, follow these best practices to ensure robust and error-free implementation:
- Always encode special characters: URL parameters may include spaces, special symbols, or non-ASCII characters. The
requests
library automatically handles encoding, but be cautious if building query strings manually. - Use a dictionary: Use a Python dictionary to define parameters so they remain structured and readable.
- Handle large parameters: For long lists or multiple parameters, consider compressing or simplifying the data to keep URLs shorter and cleaner.
- Validate API responses: Always check the status code and handle unexpected responses gracefully.
Passing Multiple Parameters
To pass multiple parameters, simply include more key-value pairs in the dictionary associated with params
. For example:
params = {'name': 'Alice', 'age': 25, 'hobby': 'reading'}
response = requests.get(url, params=params)
The resulting URL will look like:
https://api.example.com/users?name=Alice&age=25&hobby=reading
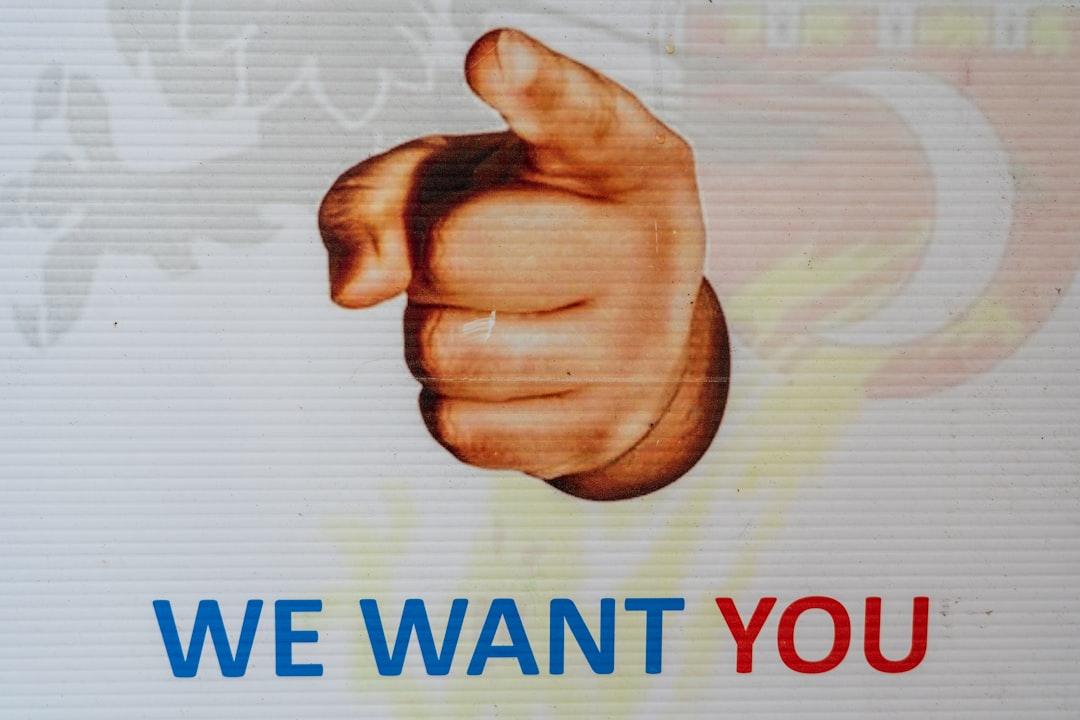
Dealing with Edge Cases
In certain situations, managing parameters can be tricky. Consider these cases:
- Handling null or empty values: Avoid adding parameters with null values as they might produce an unexpected query string.
- Preventing errors: If certain parameters are essential for the request, always validate their presence before sending the request.
- Complex nested data: If handling deeply nested data structures, use JSON instead of a query string wherever possible.
Python’s requests
library ensures parameter encoding but understanding these edge cases helps in writing robust code.
Frequently Asked Questions (FAQ)
- Q: Do I have to encode parameters manually?
A: No, therequests
library automatically encodes parameters for you. - Q: Can I pass parameters in a POST request?
A: Yes, you can pass data in thedata
parameter for a POST request, but query parameters are typically reserved for GET requests. - Q: How can I debug if my parameters are not working?
A: Print theresponse.url
to verify the final URL and check the status code of the response for errors. - Q: Can I use
requests
for APIs with authentication?
A: Yes, the library supports authentication mechanisms like Basic Auth, OAuth, and Bearer Tokens.
Mastering the concept of passing parameters into a URL using Python’s requests
library proves to be a valuable skill when working with APIs or interfacing with web services. Following the guidelines provided here ensures better usability and reduces potential issues with malformed URLs.